Java script advanced course
- Nyan Linn Aung
- May 20, 2023
- 9 min read
What is Java script?
JavaScript is a high-level, interpreted programming language primarily used for adding interactivity to web pages. It was created by Brendan Eich at Netscape Communications in 1995 and has since become one of the most popular programming languages for both web development and general-purpose application development.
JavaScript is a client-side scripting language, meaning it runs on the user's web browser rather than on a web server. This allows JavaScript to manipulate the Document Object Model (DOM), which represents the structure and content of a web page. By interacting with the DOM, JavaScript can dynamically modify the appearance and behavior of web pages in response to user actions or events.
JavaScript is often used in conjunction with HTML and CSS to create interactive web experiences. It enables developers to create features such as form validation, dynamic content updates, image sliders, interactive maps, and much more. Additionally, with the introduction of Node.js, JavaScript can also be used on the server-side to build scalable and efficient web applications.
The language itself is dynamically typed, which means variables can hold values of any data type without explicit declaration. JavaScript supports a wide range of data types, including numbers, strings, booleans, arrays, objects, and functions. It also offers powerful built-in functions and methods that can be used for various operations and manipulations.

Over the years, JavaScript has evolved with the introduction of new versions, with ECMAScript (ES) standards defining its specifications. ECMAScript 6 (ES6), released in 2015, introduced significant enhancements to the language, including arrow functions, template literals, classes, modules, and more, making JavaScript even more powerful and expressive.
JavaScript's versatility and widespread adoption have led to its usage beyond web development, with frameworks and libraries like React, Angular, and Vue.js enabling developers to build complex web applications. It is also utilized in other domains such as server-side programming, game development, desktop applications, and even Internet of Things (IoT) devices.
In summary, JavaScript is a flexible and widely used programming language that brings interactivity and dynamic functionality to web pages, allowing developers to create engaging user experiences on the web.
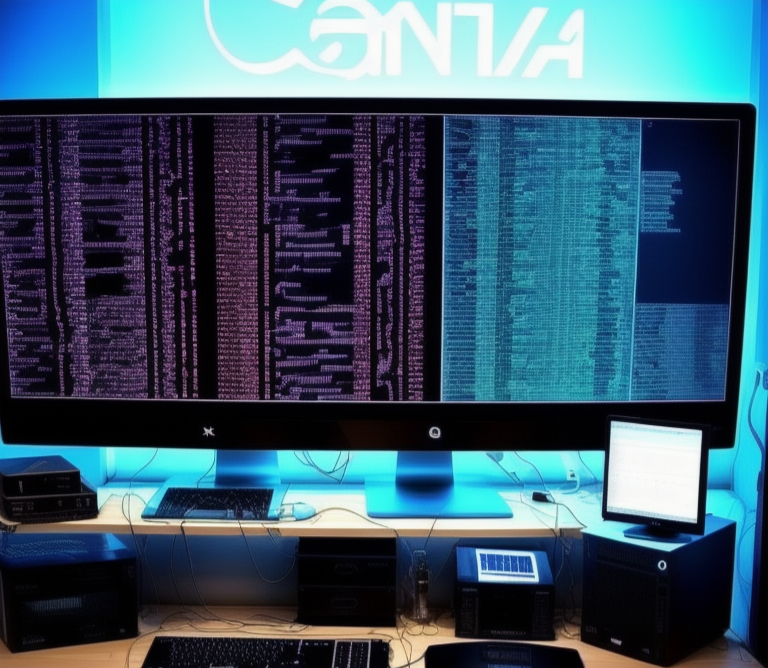
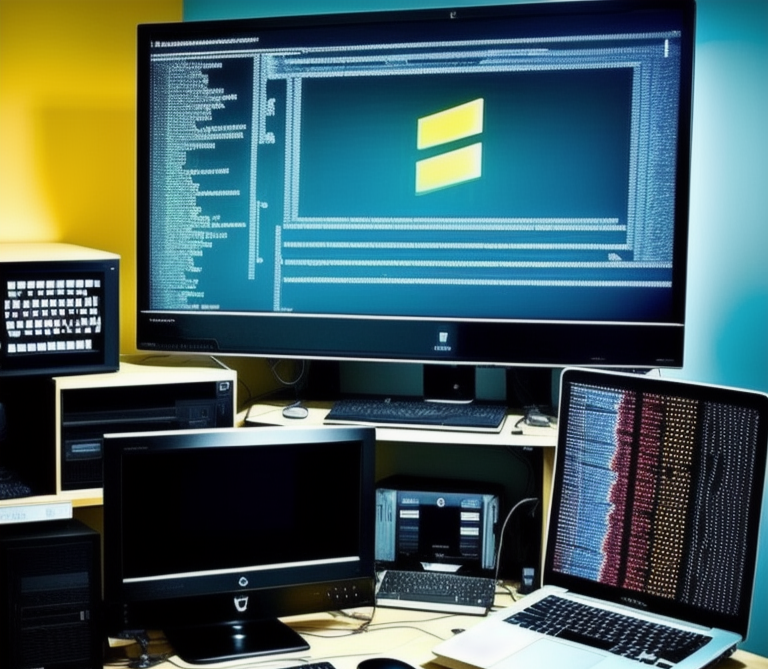
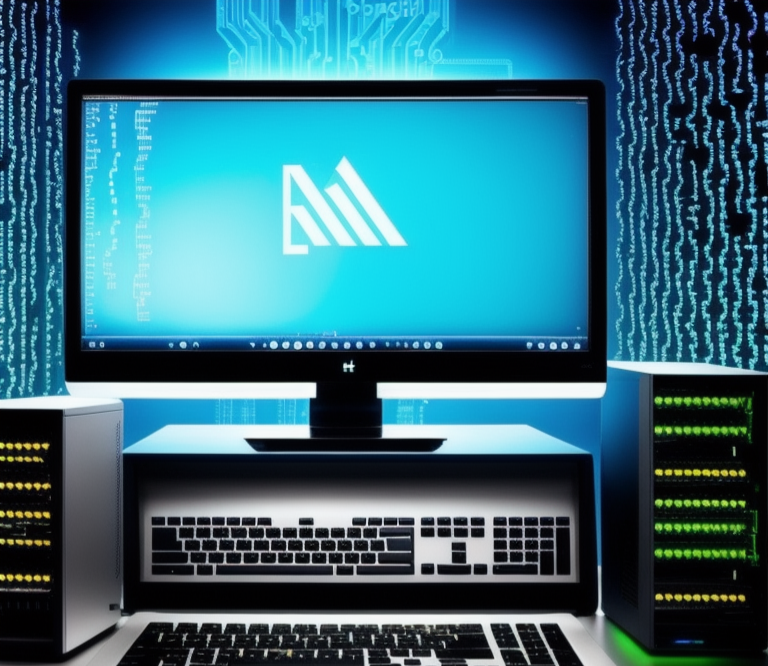
Why learn Java script?
Learning JavaScript can be incredibly beneficial for several reasons:
1. Web Development: JavaScript is an essential language for web development. It allows you to create interactive and dynamic websites by manipulating the DOM, handling user events, and making asynchronous requests. With JavaScript, you can enhance the user experience, create animations, validate forms, and build feature-rich web applications.
2. Versatility: JavaScript is not limited to just web development. It has expanded its reach to other areas such as server-side programming (with Node.js), desktop application development (using frameworks like Electron), and game development (with libraries like Phaser or Three.js). By learning JavaScript, you open up opportunities to work on diverse projects and platforms.
3. High Demand: JavaScript is one of the most widely used programming languages, making it highly in-demand in the job market. Many companies and organizations seek developers proficient in JavaScript for front-end, back-end, or full-stack development roles. Learning JavaScript can significantly enhance your career prospects and increase job opportunities.
4. Community and Resources: JavaScript has a vast and active developer community. This means there are abundant resources available, such as documentation, tutorials, forums, and libraries. You can find support, guidance, and collaboration opportunities within the JavaScript community, which can greatly facilitate your learning journey.
5. Easy to Get Started: JavaScript has a relatively low learning curve compared to other programming languages. It has a syntax similar to other C-style languages, making it accessible for beginners. You can quickly start experimenting with JavaScript code in a web browser console, without the need for complex setup or compilation processes.
6. Integration with HTML and CSS: Since JavaScript works seamlessly with HTML and CSS, learning JavaScript enhances your ability to create cohesive and interactive web experiences. You can manipulate HTML elements, dynamically style elements, and handle user interactions, resulting in engaging and responsive websites.
7. Continuous Evolution: JavaScript is continuously evolving, with regular updates and new features being introduced through ECMAScript (ES) standards. By learning JavaScript, you stay up-to-date with the latest advancements in the language, ensuring that your skills remain relevant in the ever-changing landscape of web development.
Overall, learning JavaScript provides you with a powerful and versatile toolset for web development, expands your career opportunities, and grants you access to a vibrant community and extensive resources. Whether you're a beginner or an experienced programmer, acquiring JavaScript skills is a valuable investment in your professional growth.
Setting up a development environment (Code editor & browser)
Setting up a suitable development environment for JavaScript involves choosing a code editor and a web browser. Here's a step-by-step guide to get you started:
1. Choose a Code Editor:
- Visual Studio Code (VS Code): It is a popular and highly customizable code editor with robust features and a rich extension ecosystem.
- Sublime Text: A lightweight and fast code editor that offers a smooth editing experience.
- Atom: An open-source code editor developed by GitHub, known for its hackability and extensibility.
- JetBrains WebStorm: A powerful IDE specifically designed for web development, offering advanced features and intelligent code suggestions.
2. Install the Code Editor:
- Visit the official website of your chosen code editor.
- Download the installer suitable for your operating system (Windows, macOS, or Linux).
- Run the installer and follow the on-screen instructions to complete the installation process.
3. Configure the Code Editor:
- Launch the code editor.
- Explore the preferences or settings to customize the editor to your liking.
- Install helpful extensions or plugins for JavaScript development (e.g., linters, formatters, debuggers) through the editor's extension marketplace.
4. Choose a Web Browser:
- Google Chrome: Widely used and offers powerful development tools.
- Mozilla Firefox: A popular browser with excellent developer tools and privacy features.
- Microsoft Edge: The default browser on Windows, which also provides solid development tools.
- Safari: The default browser on macOS and iOS devices, essential for testing on Apple platforms.
5. Install Web Browsers:
- Visit the official websites of your chosen web browsers.
- Download the browser installers suitable for your operating system.
- Run the installers and follow the on-screen instructions to complete the installation.
6. Test and Debug:
- Open your code editor and create a new JavaScript file.
- Write a simple script to test the setup, such as displaying a "Hello, World!" message in the browser's console.
- Save the file with a .js extension (e.g., script.js).
- Open your preferred web browser and launch its developer tools.
- In the browser's developer tools, navigate to the "Console" tab.
- Link the JavaScript file to an HTML file using a `<script>` tag or use the browser's console to execute JavaScript directly.
- Verify that the "Hello, World!" message or any other output appears in the browser's console without any errors.
Congratulations! You have set up your development environment with a code editor and a web browser. Now you can start writing, testing, and debugging JavaScript code to build web applications or explore JavaScript concepts further.
Remember to keep your code editor and web browser updated to benefit from the latest features and improvements. Additionally, consider using version control systems like Git to track changes in your code and collaborate effectively with others.
Step 2- Variables and Data types
Declaring variables using 'let' and 'const'
In JavaScript, you can declare variables using two keywords: `let` and `const`. These keywords have slightly different behaviors and use cases. Here's an overview of how to use them:
1. `let`:
- The `let` keyword is used to declare variables that can be reassigned.
- You can declare a variable using `let` and optionally assign an initial value to it.
- Example:
```javascript
let name;
name = "John"; // Variable assignment
let age = 25; // Variable declaration and assignment in one line
name = "Jane"; // Variable reassignment
```
2. `const`:
- The `const` keyword is used to declare variables that are constant, meaning their values cannot bebp
- You must assign an initial value to a `const` variable when declaring it.
- Example:
```javascript
const pi = 3.14159; // Constant variable declaration and assignment
/bpi = 3.14; // Error: Assignment to a constant variable is not allowed
```
3. Best Practices:
- Use `let` when you need a variable that may change its value during the program's execution.
- Use `const` when you have a value that should remain constant and not be reassigned.
- It is generally recommended to use `const` whenever possible, as it promotes immutability and helps prevent accidental variable reassignment.
4. Scoping:
- Both `let` and `const` have block scope, meaning they are accessible only within the block (within curly braces) where they are defined.
- If you declare a variable with `let` or `const` inside a function, it will be limited to that function's scope.
- Example:
```javascript
function exampleFunction() {
let x = 10; // x is only accessible within this function
if (x > 5) {
const y = 20; // y is only accessible within this if block
console.log(x + y); // Output: 30
}
// console.log(y); // Error: y is not accessible here
}
```
Remember to choose the appropriate keyword (`let` or `const`) based on whether you need a variable that can be reassigned or a constant value. This helps maintain code clarity and prevents unintended changes to variable values.
Primitive data types (numbers, string, boleans)
In JavaScript, there are three primitive data types: numbers, strings, and booleans. These data types represent the most basic and fundamental values in the language. Here's an overview of each primitive data type:f
1. Numbers:
- The number data type represents numeric values, both integers and floating-point numbers.
- Example:
```javascript
let age = 25; // Integer
let pi = 3.14; // Floating-point number
```
2. Strings:
- The string data type represents textual data, enclosed in single quotes (''), double quotes ("") or backticks (``).
- Example:
```javascript
let name = 'John'; // Single quotes
let message = "Hello, world!"; // Double quotes
let template = `My name is ${name}.`; // Backticks for template literals
```
3. Booleans:
- The boolean data type represents logical values: `true` or `false`.
- Booleans are often used in conditions and comparisons to control the flow of programs.
- Example:
```javascript
let isAdult = true;
let isStudent = false;
```
These primitive data types are immutable, which means their values cannot be changed once assigned. However, you can reassign variables with new values of the same or different data type.
JavaScriptJavaScript also provides some built-in functions and methods for working with primitive data types. For example, you can use methods like `toString()` and `length` to manipulate strings, and operators like `+`, `-`, `*`, `/`, and `%` to perform arithmetic operations on numbers.
Understanding and working with these primitive data types is foundational to writing JavaScript code. They allow you to store and manipulate different kinds of values to perform calculations, display information, and make logical decisions within your programs.
Working with arrays and objects.
In JavaScript, arrays and objects are powerful data structures that allow you to store and organize related data. They provide flexibility and versatility in managing collections of values and properties. Let's explore working with arrays and objects in JavaScript:
1. Arrays:
- An array is an ordered collection of values enclosed in square brackets ([]).
- Each value within an array is called an element, and it can be of any data type.
- Example:
```javascript
let numbers = [1, 2, 3, 4, 5]; // Array of numbers
let fruits = ['apple', 'banana', 'orange']; // Array of strings
let mixed = [true, 'hello', 42]; // Array of mixed data types
```
- Accessing Elements: You can access individual elements within an array using square bracket notation, starting with an index of 0.
```javascript
console.log(numbers[0]); // Output: 1
console.log(fruits[2]); // Output: orange'''
`
- Modifying Elements: You can modify elements within an array by assigning new values to specific indices.
```javascript
numbers[3] = 10; // Modifying an element
fruits[1] = 'grape'; // Modifying an element
```
- Array Methods: JavaScript provides various built-in methods to manipulate arrays, such as `push()`, `pop()`, `shift()`, `unshift()`, `slice()`, `splice()`, and more. These methods allow you to add, remove, and modify elements within an array easily.
2. Objects:
- An object is an unordered collection of key-value pairs, enclosed in curly braces ({}).
- Each key represents a property name, and its associated value can be of any data type.
- Example:
```javascript
let person = {
name: 'John',
age: 25,
isStudent: true
};
let book = {
title: 'JavaScript Basics',
tutor : 'Jane Doe',
year: 2022
};
```
- Accessing Properties: You can access properties within an object using dot notation or square bracket notation.
```javascript
console.log(person.name); // Output: John
console.log(book['author']); // Output: Jane Doe
```
- Modifying Properties: You can modify properties within an object by assigning new values to them.
```javascript
person.age = 30; // Modifying a property
book.title = 'JavaScript Advanced'; // Modifying a property
```
- Object Methods: Objects can have associated functions, called methods, which can be invoked using dot notation. Methods allow objects to perform actions or computations.
```javascript
let calculator = {
add: function (a, b) {
return a + b;
},
multiply: function (a, b) {
return a * b;
}
};
console.log(calculator.add(5, 3)); // Output: 8
console.log(calculator.multiply(2, 4)); // Output: 8
```
- Object Iteration: You can iterate over an object's properties using loops like `for...in` or `Object.keys()` to access and manipulate each property.
Arrays and objects are extensively used in JavaScript to store and organize data in various scenarios. They offer flexible ways to represent complex structures and enable powerful data manipulation and retrieval operations.
Comentarios