Android Studio
- Nyan Linn Aung
- May 31, 2023
- 5 min read
Title: Mastering Android Studio: The Ultimate Guide for App Developers
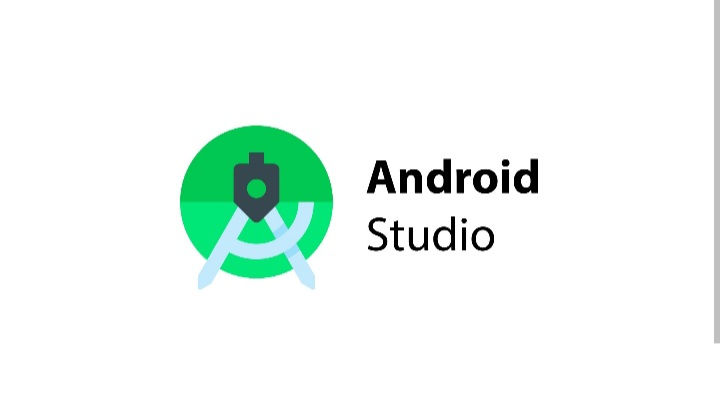
Introduction:
Welcome to the ultimate guide for mastering Android Studio! As the official integrated development environment (IDE) for Android app development, Android Studio offers a wide range of powerful tools and features to help you create exceptional Android applications. Whether you're a beginner or an experienced developer, this comprehensive guide will walk you through each aspect of Android Studio, providing detailed explanations and step-by-step instructions to help you become proficient in building Android apps.
Chapter 1: Understanding Android Studio
1.1 What is Android Studio?
- Introduction to Android Studio and its role in Android app development.
- Evolution and history of Android Studio.
1.2 Why Use Android Studio?
- Advantages of using Android Studio over other development tools.
- Integration with Android SDK and Google services.
- Support for Kotlin, the modern programming language for Android development.
1.3 Key Features of Android Studio
- Overview of essential features such as code editor, layout editor, and debugging tools.
- Profiling and performance analysis capabilities.
- Integration with version control systems and collaboration tools.
Chapter 2: Getting Started with Android Studio
2.1 Installing Android Studio
- Step-by-step installation guide for Windows, macOS, and Linux.
- Configuring system requirements and hardware acceleration.
2.2 Configuring SDK and Emulators
- Setting up Android SDK and configuring API levels.
- Creating and managing virtual devices (emulators) for testing apps.
2.3 Tour of the Android Studio Interface
- Introduction to the different panels, toolbars, and menus in Android Studio.
- Exploring the project structure and files.
Chapter 3: Creating a New Project
3.1 Project Structure in Android Studio
- Understanding the directory structure of an Android project.
- Exploring the purpose and contents of essential files.
3.2 Choosing Project Templates
- Overview of various project templates available in Android Studio.
- Selecting the appropriate template for your app's requirements.
3.3 Working with Modules and Dependencies
- Creating and managing modules within a project.
- Adding external libraries and dependencies to enhance app functionality.
Chapter 4: Exploring the Code Editor
4.1 Understanding the Code Editor Layout
- Introduction to the code editor interface and its components.
- Customizing the editor settings and preferences.
4.2 Navigating and Searching in Code
- Efficient navigation techniques within the codebase.
- Utilizing the search functionality to find classes, methods, and resources.
4.3 Code Formatting and Code Analysis
- Applying code formatting rules to maintain a consistent coding style.
- Utilizing code analysis tools to identify and fix potential issues.
Chapter 5: Building User Interfaces
5.1 Layout Editor in Android Studio
- Designing app layouts visually using the layout editor.
- Drag-and-drop functionality and properties adjustment.
5.2 Working with Views and Widgets
- Introduction to Android views and widgets.
- Adding and customizing UI elements using XML and code.
5.3 XML Layout Files and Resource Management
- Understanding the structure and syntax of XML layout files.
- Managing resources such as strings, colors, and dimensions.
Chapter 6: Managing App Resources
6.1 Understanding Android Resources
- Overview of Android resources and their importance.
- Utilizing resource qualifiers for different screen sizes and languages.
6.2 Managing Strings, Colors, and Dimensions
- Storing and accessing strings, colors, and dimensions in resources.
- Using resource files for localization and theming.
6.3 Localization and Internationalization
- Localizing your app for different languages and regions.
- Implement
ing internationalization features to adapt app content dynamically.
Chapter 7: Working with Activities and Fragments
7.1 Creating Activities and Fragments
- Introduction to activities and fragments in Android.
- Creating and managing multiple screens in your app.
7.2 Navigation and Backstack Management
- Implementing navigation between activities and fragments.
- Managing the backstack and handling navigation transitions.
7.3 Activity Lifecycle and State Management
- Understanding the lifecycle of an activity and fragment.
- Saving and restoring instance state for preserving data.
Chapter 8: Data Persistence and Storage
8.1 Working with SQLite Databases
- Creating and managing SQLite databases for local data storage.
- Executing queries and performing CRUD operations.
8.2 Using SharedPreferences for Data Storage
- Storing app preferences and small data sets using SharedPreferences.
- Retrieving and updating SharedPreferences values.
8.3 Integrating Content Providers
- Using content providers to share data across multiple apps.
- Implementing custom content providers for app-specific data sharing.
Chapter 9: Working with Network APIs
9.1 Consuming RESTful APIs
- Making HTTP requests to consume data from RESTful APIs.
- Handling responses and parsing JSON or XML data.
9.2 Making HTTP Requests with Retrofit
- Overview of Retrofit library for simplified network requests.
- Defining API endpoints and performing asynchronous requests.
9.3 Parsing JSON Responses with Gson
- Using Gson library to parse JSON responses into Java/Kotlin objects.
- Mapping JSON data to model classes.
Chapter 10: Debugging and Testing
10.1 Debugging Techniques in Android Studio
- Utilizing breakpoints, watches, and logs for debugging.
- Inspecting variables and analyzing app behavior.
10.2 Unit Testing with JUnit and Mockito
- Writing and executing unit tests for your app logic.
- Mocking dependencies using Mockito.
10.3 UI Testing with Espresso
- Automating user interface testing using Espresso.
- Writing UI tests for app interactions and verifications.
Chapter 11: Performance Optimization
11.1 Profiling and Analyzing App Performance
- Profiling app performance using Android Studio's built-in tools.
- Identifying and optimizing performance bottlenecks.
11.2 Memory Management and Optimization
- Understanding memory management in Android.
- Implementing strategies to optimize memory usage.
11.3 Optimizing Network Calls and Battery Usage
- Efficiently handling network requests to minimize battery drain.
- Implementing caching and optimizing network calls.
Chapter 12: Integrating Libraries and SDKs
12.1 Adding External Libraries and Dependencies
- Integrating third-party libraries into your Android project.
- Managing dependencies using Gradle.
12.2 Working with Google Play Services
- Utilizing Google Play Services APIs for maps, location, and more.
- Configuring and integrating required libraries.
12.3 Implementing In-App Billing and Advertisements
- Monetizing your app by integrating in-app billing functionality.
- Displaying ads in your app using Google AdMob.
Chapter 13: Version Control and Collaboration
13.1 Using Git for Version Control
- Setting up Git for version control in Android Studio.
- Performing essential Git operations, such as commit, branch, and merge.
13.2 Collaborating with Other Developers
- Working in a team environment using Git branches.
- Resolving merge conflicts and maintaining code integrity.
13.3 Branching and Merging Strategies
- Understanding different branching and merging strategies for efficient collaboration.
- Choosing the appropriate strategy for your development workflow.
Chapter 14: Publishing and Distribution
14.1 Preparing for App Release
- Finalizing app features and functionality before release.
- Optimizing app performance and ensuring quality.
14.2 Generating Signed APKs
- Creating signed APKs for release distribution.
- Configuring app signing and security measures.
14.3 Uploading Apps to Google Play Store
- Preparing app assets and metadata for Google Play Store submission.
- Uploading APKs and managing app listings.
Chapter 15: Advanced Topics and Best Practices
15.1 Kotlin Programming in Android Studio
- Exploring advanced Kotlin features and language constructs.
- Leveraging Kotlin's advantages for Android development.
15.2 Architectural Patterns (MVC, MVP, MVVM)
- Understanding different architectural patterns for app development.
- Implementing Model-View-Controller (MVC), Model-View-Presenter (MVP), and Model-View-ViewModel (MVVM) patterns.
15.3 Accessibility and Localization Guidelines
- Ensuring accessibility compliance for users with disabilities.
- Implementing localization best practices for app global reach.
Chapter 16: Troubleshooting and Resources
16.1 Common Issues and Error Handling
- Identifying and resolving common issues faced during Android development.
- Handling runtime errors and exceptions.
16.2 Android Studio Documentation and Community Support
- Leveraging official Android Studio documentation for reference and learning.
- Engaging with the Android developer community for support and guidance.
16.3 Online Resources and Learning Platforms
- Discovering additional online resources, tutorials, and courses for further learning.
- Exploring platforms and forums for discussing and sharing Android development knowledge.
Conclusion:
Congratulations on completing this comprehensive guide to mastering Android Studio! With the knowledge gained from this guide, you're equipped to create remarkable Android applications with efficiency and confidence. Android Studio's rich set of tools and features, combined with your skills, will enable you to bring your app ideas to life and make a positive impact in the Android ecosystem.
Remember, practice and continuous learning are crucial for honing your Android development skills. Stay up-to-date with the latest advancements in Android Studio and explore new features and libraries to enhance your app development process. Engage with the Android developer community, seek guidance, and contribute to the growth of the community.
Now, armed with the expertise gained from this guide, embark on your Android development journey and create innovative and impactful apps using Android Studio. Happy coding!
Comments